1. Overview
This portfolio documents my contributions towards the UltiStudent project which is part of an introductory software engineering module known as CS2103T - Software Engineering. During this module, we have been provided with a working software application that could enhance and morph our application to suit our target audience.
The purpose of this document is to present the principles and practical skills I have learnt throughout the course of this module.
2. PROJECT: UltiStudent
UltiStudent is a student application that effectively tackles the problem of having too many applications to manage their school life. Having a large number of different platforms can lead to inconvenience, incompatibilities and inorganisation. UltiStudent tackles this problem by providing a centralised platform for them to manage their homework, Cumulative Average Point (CAP) and notes to improve the quality of their school life.
UltiStudent provides a Command Line Interface (CLI) for students to interact with and is complemented by a Graphical User Interface (GUI). We have designed the application with the user in mind.
3. Summary of contributions
This section contains the key contributions I have made towards this project. They highlight my ability to |
-
Major Enhancement:
-
Developed the
Model
,Logic
andStorage
infrastructure for the Homework Manager.-
Functionality: Allows the user to
add
,delete
,edit
,find
andlist
in the homework entries. Provides the foundations on which these features are built. -
Justification: The ability to create homework entries and other features such as edit depends on the Homework Manager infrastructure. This enhances the features of the UltiStudent by allowing students to manage their homework on our application.
-
Highlights: This enhancement is a core component of our application. It affects the current functionalities of our application and the future extensions of our application. To do this well, it requires an in-depth understanding of the initial infrastructure of the software in order to alter it. The implementation was also difficult because of the various abstraction layers that had to be understood.
-
-
-
Minor enhancement:
-
Edit Homework command that edits the attributes of current existing homework entries based on user input.
-
Delete Homework command that deletes the attributes whose note name has the keywords indicated by the user.
-
Find Module command that filters all the homework entries based on a list of modules.
-
List Homework command that shows the entire list of homework entries in the Homework Manager.
-
-
Code contributed: Click here to view my code on the CS2103T Project Code Dashboard.
-
Other contributions:
-
Project management:
-
Helped to manage issue tracker for our project repository.
-
Planned the project milestones for the Homework Manager feature of UltiStudent.
-
-
Enhancements to existing features:
-
Documentation:
-
User Guide
-
Revamped the tone of the User Guide to make it sound more friendly.
-
-
Developer Guide
-
Created UML diagrams to better explain the
edit-hw
andfind-mod
commands in the Developer Guide. -
Discussed the pros and cons of different implementations considered during the design stage.
-
-
-
Community:
-
Contributed to forum discussions. #25
-
-
Tools:
-
Assisted teammate in the integration of the Travis CI into our repository.
-
-
{you can add/remove categories in the list above}
4. Contributions to the User Guide
The following are the contributions I have made to the User Guide. They demonstrate my ability to write clear and concise documentation in friendly and inviting manner targeted at non-technical users. |
4.1. Editing a homework: edit-hw
Format: edit-homework INDEX [mc/MODULECODE] [hw/HOMEWORK] [d/DEADLINE]
Realised that you made a mistake in an entry after you added it? Introducing our edit command!
You can make any changes to any of the attributes of the homework entry using this command!
To use this command, we must follow this format: edit-homework INDEX [mc/MODULECODE] [hw/HOMEWORK]
[d/DEADLINE]
You can refer to the diagram below to understand what we mean by the word index
!
You may have noticed that it looks rather similar to the add-hw command. Good observation!
However, you should take note of the slight difference that you do not have to include all the attributes.
Instead, only include those you wish to change. Any other attribute(s) is optional.
Now let’s take a look at an example so that you can understand what I mean more clearly.
So you already have a homework entry in the homework manager which has these
mc/CS1101S hw/Tutorial 1 d/01/05/2019
as its attributes.
Now let’s say our professor has decided to extend the deadline by a week upon requests by students.
Simply type edit-hw 1 d/08/05/2019
and the update will be reflected in your homework entry.
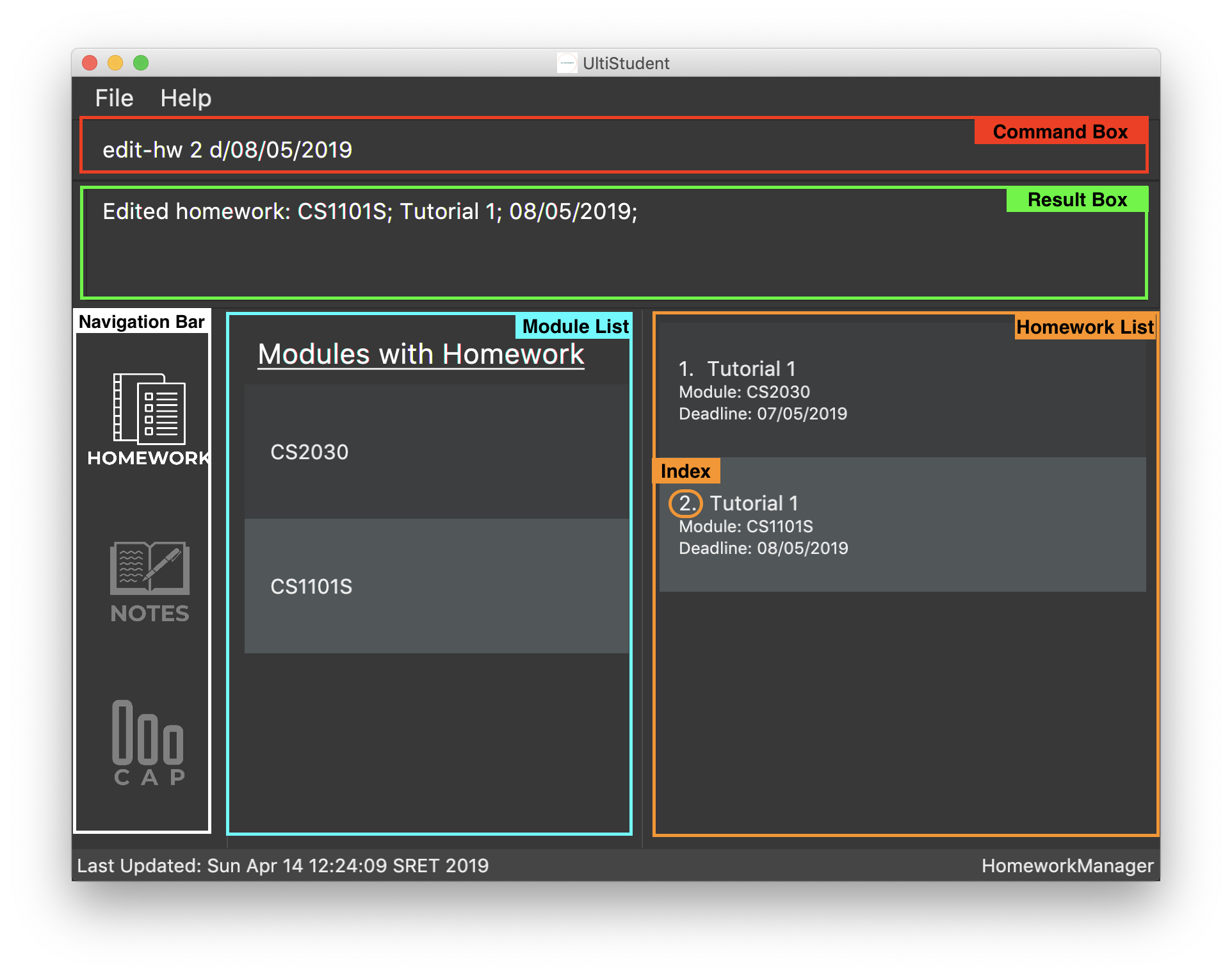
After entering your edit-hw
command, the message you see in the green highlighted box
in the diagram above indicates that you have successfully done it.
You can also see how the deadline of the attribute has been updated according to the deadline you wish to edit it to.
You can edit any amount of attributes at for one homework entry at one time! Include all the attributes you want to alter based on the format and exclude any other attributes you do not want to change. |
4.2. Deleting a homework: delete-hw
Format: delete-hw INDEX
Now let’s say you are done with your homework and you wish to remove that entry from the homework manager.
You can easily delete it by using the delete-hw
command!
All you have to do is to follow this short and simple format delete-hw INDEX
.
Using the same example in the edit command. Let’s say we are done with the CS1101S homework
and wish to remove it from the Homework Manager. All you have to do is to type delete-hw 2
and you are done!
Our delete command is the shortest command in UltiStudent and the easiest to use.
If you happen to forget what we mean by the word INDEX
,
simply refer to the diagram in the edit-hw section (Section 4.2.2) to understand what it means!
4.3. Finding homework from a specified module: find-mod
Format: find-mod [KEYWORDS]
As we get later into the semester, there will be more work for us to do. Every single module has some form of submission and you will soon have so many entries in your homework list that it makes it difficult to read and find a particular entry. We have a special command for you to use and find all the homework entries belonging to a specific module(s).
The format of this command is simple. find-mod [KEYWORDS]
.
As you can see from the diagram below, after adding a module code as part of a keyword,
you will be able to see only homework from the module in the homework list.
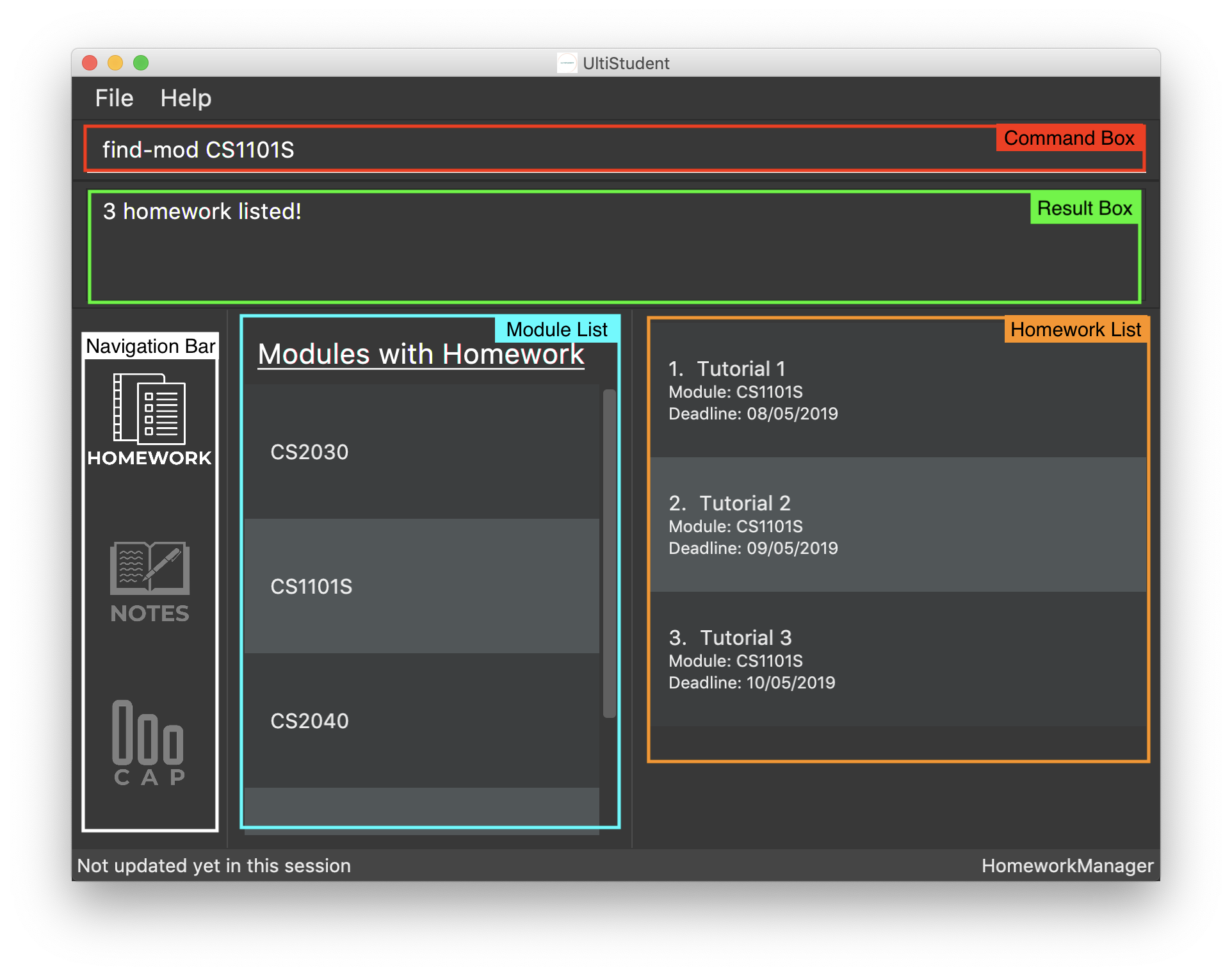
As you can see from the diagram above, even though there are a total of four modules with homework in the module list,
only homework from the modules CS1101S is shown after the common find-mod CS1101S
is used.
You are not restricted to only one module code for the [KEYWORDS] in the command.
You can add more of them if you wish.
|
4.4. Reverting back to a full list view: list-hw
Format: list-hw
In the previous sub-section, we looked at how we can use the command find-mod
to display
homework entries from selected module codes. Now, you are probably wondering now how to display a full list of modules.
To display the full list, simply use this command list-hw
. This command has no additional inputs for you to type
and that makes this simple and easy to use!
5. Contributions to the Developer Guide
The following are the contributions I have made to the Developer Guide. They demonstrate my ability to present and explain complicated technical information in a comprehensible manner. It also showcases my technical skills and abilities. |
5.1. Delete homework feature
This feature allows the user to delete a homework entry from the homework manager through its index.
The delete homework feature is facilitated by the DeleteHomeworkCommandParser
and the DeleteHomeworkCommand
.
The delete command is part of the logic component of our application. It interacts with the model and the storage components of our application.
5.1.1. Overview
The DeleteHomeworkCommandParser
implements Parser
with the following operation:
-
DeleteHomeworkCommandParser#parse()
- This operation will take in aint
input from the user which will delete the homework entry at the index which has entered. Any invalid format of the command will be prompted by the command parser.
5.1.2. Current Implementation
The delete homework feature is executed by the DeleteHomeworkCommand
. Currently, the
deletion of any homework entry is done based on the INDEX
of the homework entry.
During the design of our delete function, we considered between two alternatives.
Design Consideration | Pros and Cons |
---|---|
Deletion by Index (Current Choice) |
Pros: Since each homework has a unique index, any deletion by the index is less prone to bugs and easier to implement. Cons: User will have to scroll for the homework entry and look for its index which can be inconvenient |
Deletion by Homework Name |
Pros: It may be more intuitive for users to delete a homework through the name of the homework. Cons: Homework for different modules can have different names. For example, two different homework entries for two different modules can be called 'Tutorial 1'. |
We have decided to opt for the first option primarily because it reduces the number of potential bugs and the complexities involved when taking into account the different cases upon using deletion by homework name.
5.2. Edit homework feature
This feature allows the user to edit any attribute of the homework entries. This is a core feature because the user may have to update deadline or make corrections to mistakes, such as typographical errors, when adding a homework entry. There are a total of three attributes for each entry, the module code, the homework name and the deadline. The user can edit at least one and up to three attributes.
The edit homework feature is facilitated by the EditHomeworkCommandParser
and the EditHomeworkCommand
.
The edit command is part of the logic component of our application. It interacts with the model component of our software architecture.
5.2.1. Overview
The EditHomeworkCommandParser
implements Parser
with the following operation:
-
EditHomeworkCommandParser#parse()
- This operation will take in anint
input and one to threeString
input(s) from the user that will alter the attributes of current homework entries based on the prefixes 'mc/', 'hw/' and 'd/'. TheString
value after the individual prefixes will alter the respective attribute it corresponds to: 'mc/' forModuleCode
,'hw/' forHomeworkName
and 'd/' forDate
. A validation check will be imposed upon editing of each object. Any checks that fails the validation would prompt the user on the failed component.
5.2.2. Current Implementation
Here is an example of a step-by-step process on how the edit command alters an attribute of the homework entry.
For each step, you may follow the activity diagram at the end of this section to better understand the flow of events
within UltiStudent when an edit-hw
command is inputted.
Step 1. The user launches the application and opens the homework manager. There is a list of existing homework entries in the homework manager.
Step 2. The user then wishes to alter the homework deadline of the third entry in the homework list to 10 May 2019.
He then types edit-hw d/10/05/2019
into the Command Line Interface (CLI) and executes it.
Step 3. The UltiStudentParser (refer to logic) then reads in these attributes that have been inputted and proceeds to alter the attributes of the homework entry in the given index. Each attribute will be validated.
Step 4. The UltiStudentParser then creates a new EditHomeworkCommand based on the input of the user. When the EditHomeworkCommand is executed, it interacts with the Model architecture by calling the setHomework method. The setHomework method replaces the current homework entry with the a new homework entry containing all the desired attributes. The homework entry is now updated.
Step 5. If the module code for any homework entry has been edited to a module code that is not in the module code list, the EditHomeworkCommand will add the new module code into the module code list. The updated module code list will be displayed on our User Interface.
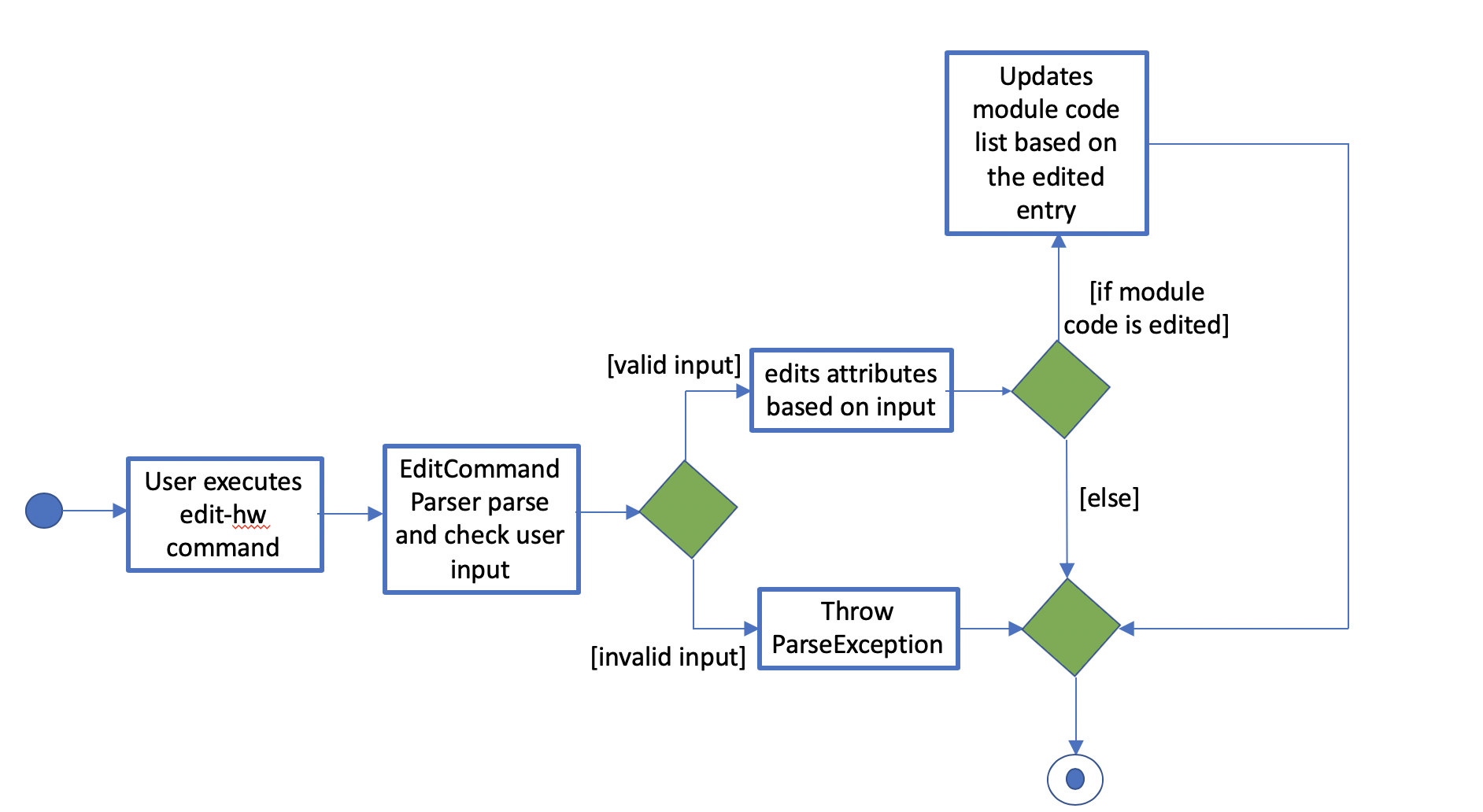
edit-hw
In designing the edit homework feature, we considered if we should use an alternative data structure to update the list of module codes. We considered using a hash map to map the module code to the number of homework with the modules to check if the module code list has to be updated for each edit.
Design Consideration | Pros and Cons |
---|---|
Update ModuleList by iteration (Current Choice) |
Pros: Protects the abstraction layers and modularity by restricting the usage to the existing data structures already present in our code. Cons: Less efficient in terms of time and actual time taken can be long when number of entries is large. |
Update ModuleList using help from other data structures. |
Pros: Faster expected performance. Cons: Introducing a new data structure can disrupt the existing abstractions of our code. |
To sum up our justification of our choice of design, we decided to opt for the first option because we prioritised the existing design abstractions in our code over the efficiency of our code.
5.3. Find module feature
This feature allows the user to only display homework belonging to one or more module codes which is user has specified. The find module feature is exceptionally useful to shorten the displayed list of homework.
The find module feature is facilitated by the FindModuleCommandParser
and the FindModuleCommand
.
It interacts with the model component of our architecture to retrieve the list homework.
5.3.1. Overview
The FindModuleCommandParser
implements Parser
with the following operation:
-
FindModuleCommandParser#parse()
- This operation will take in at least oneString
input from the user and display any of the homework entries with matches any of list of[KEYWORDS]
that the user has inputted. Each keyword is separated by a white space. Any homework entry that matches with the list of keywords will be displayed on the homework list.
5.3.2. Current Implementation
The find module feature is facilitated by the FindModuleCommand
.
Here is a sequence of steps on how the find module feature works. We will use an example here to help you understand the flow of events. In the steps below, we will make references to the diagram at the end of the section to help you visualise the sequence of events better.
Step 1. The user first launches the application and enters the homework manager. There are already existing homework entries within the homework list.
Step 2. Currently, there are a total of twelve homework entries belonging to four modules.
(Do note that this is an arbitrary state of the storage in the homework manager and is meant to serve as an example.)
Now the user inputs find mod CS1101S
. Referring to the diagram below, UltiStudentCommandParser
parses the command find-mod
together with the list of keywords.
Step 3. The FindModuleParser then creates a FindModuleCommand and then returns it to the LogicManager, which are illustrated by the blue arrows pointing from the command parsers back to the LogicManger.
Step 4. Now, the Logic Manager then executes this command. When the find command is executed, it called the method updateFilteredList, which then returns a list of homework that has the module codes which matches any of the key words inputted back to the Logic Manager as indicated by the purple arrows pointing back to the Logic Manager’s blue block.
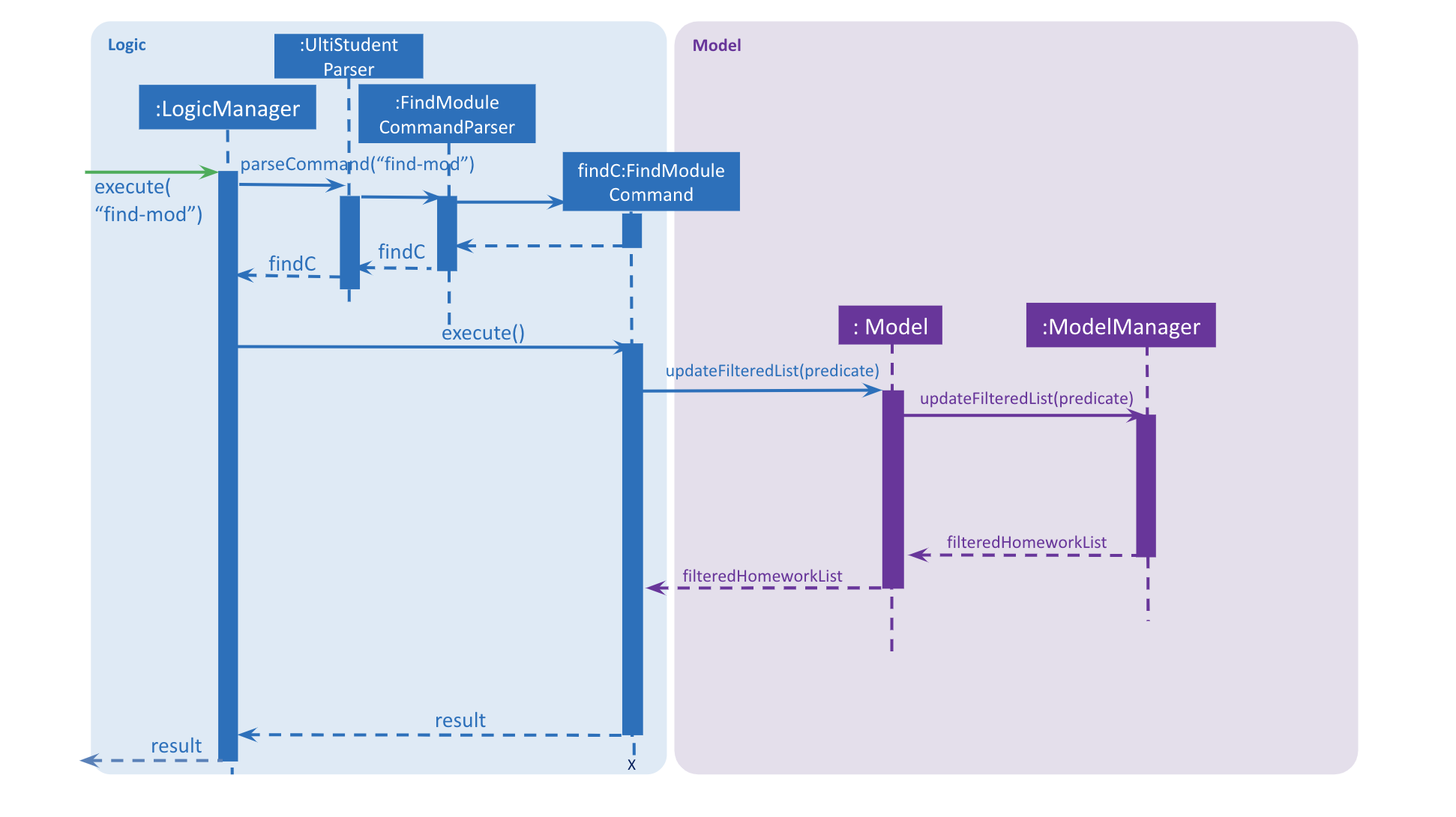
find-mod