Introduction
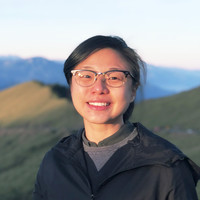
[GitHub]
Hi there! I am Shi Mei, a Year 2 Undergraduate studying in National University of Singapore (NUS) Computer Science.
The purpose of this portfolio is to document my contributions to UltiStudent, a software engineering project under CS2103T Software Engineering module in NUS.
This project (UltiStudent) would not be possible without my amazing team mates: Andrew, Benjamin, and Xavier.
1. Overview of UltiStudent
UltiStudent is a student application that effectively tackles the problem of having too many applications to manage their school life. To improve the quality of student life in computing, UltiStudent provides a centralised platform for them to manage their homework, cumulative average point (CAP) and notes.
Main Features of UltiStudent:
-
Keep track of your deadlines from various modules (Homework Manager)
-
Easy creation of digital notes (Notes Manager)
-
Projection and Prediction of CAP (CAP Manager)
…without leaving the application!
2. Summary of contributions
This section is to provide a summary of my contributions to the project.
My main contribution for UltiStudent is the creation of the Notes Manager.
The photo below shows the homepage of the Notes Manager.
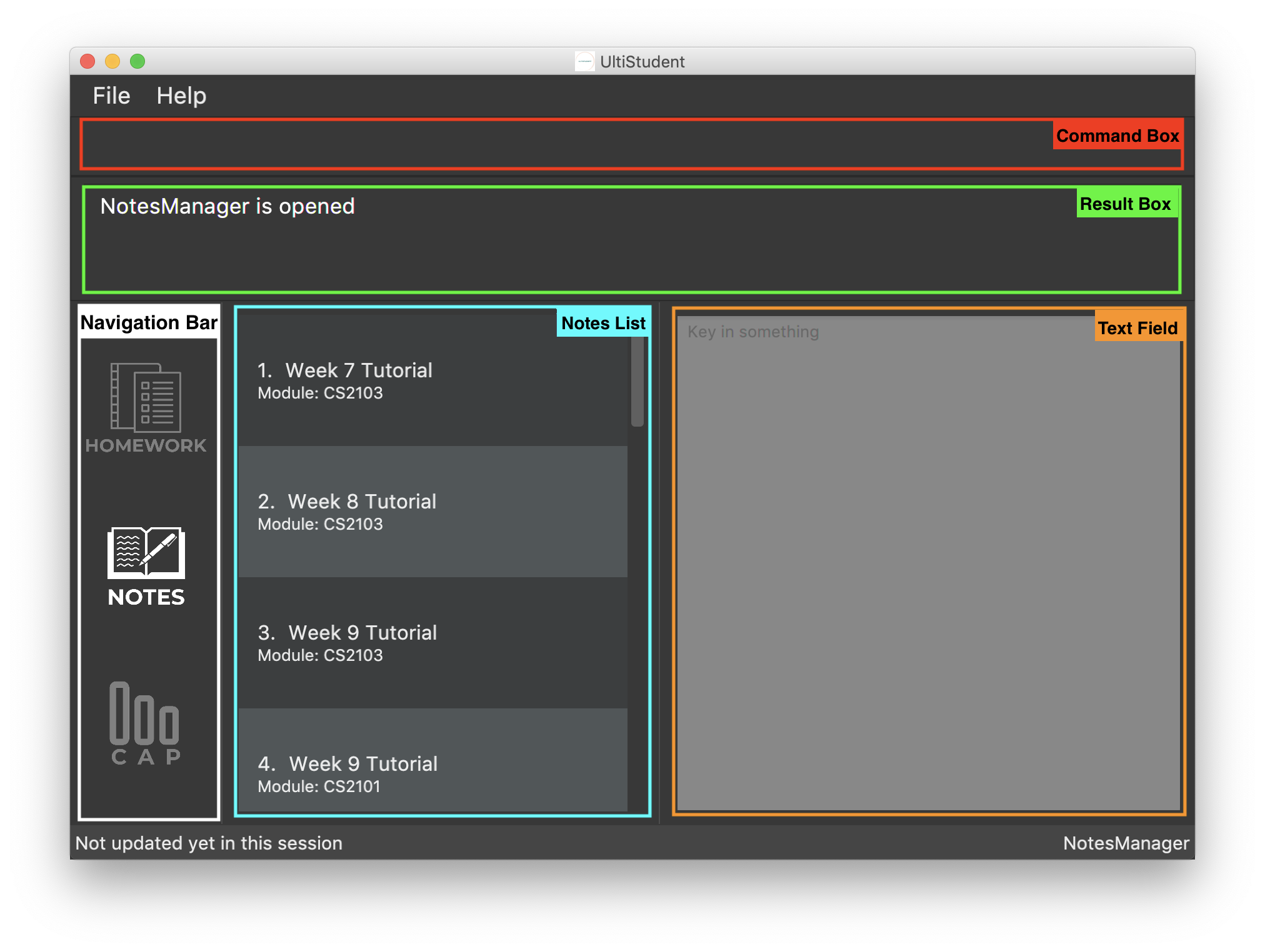
Notes Manager allows users of UltiStudent to: Add and delete notes, save and edit the content of their notes, find notes easily, list all their notes.
I was in charge of Add, Delete, Find, and List note commands.
-
Major enhancement: Developed the logic, storage, and model components of the Notes Manager infrastructure.
-
What it does: allows the user to create and delete notes from the Notes Manager.
-
Justification: The ability to create notes depends on the Notes Manager infrastructure. This improves the product significantly by allowing the addition of other features such as Find, List, Edit, and Save.
-
Highlights: This enhancement affects existing commands and commands to be added to the Notes Manager in the future. It required an in-depth analysis of design alternatives. The implementation too was challenging as it required changes to existing commands.
-
-
Minor enhancement:
-
Find note command that lists all notes whose note name has the keywords indicated by the user.
-
List note command that lists all notes in the Notes Manager.
-
-
Code contributed: RepoSense
-
Other contributions:
3. Contributions to the User Guide
This section is to showcase my main contribution to the User Guide, and highlight my ability to write documentation targeting end-users.
3.1. Adding a note : add-note
Imagine yourself sitting in CS2103T Week 7 lecture, and the Professor have just covered an important concept which you would like to take some notes on. Let Notes Manager handle that for you by storing your notes digitally for you!
To use this command we have to follow this format: add-note mc/MODULECODE
n/NOTENAME
First, we will need to be in the Note Manager. To open Notes Manager,
enter open Notes Manager
into the Command Box (red). You will see the
Result Box (green) prompting that the Notes Mananger is opened.
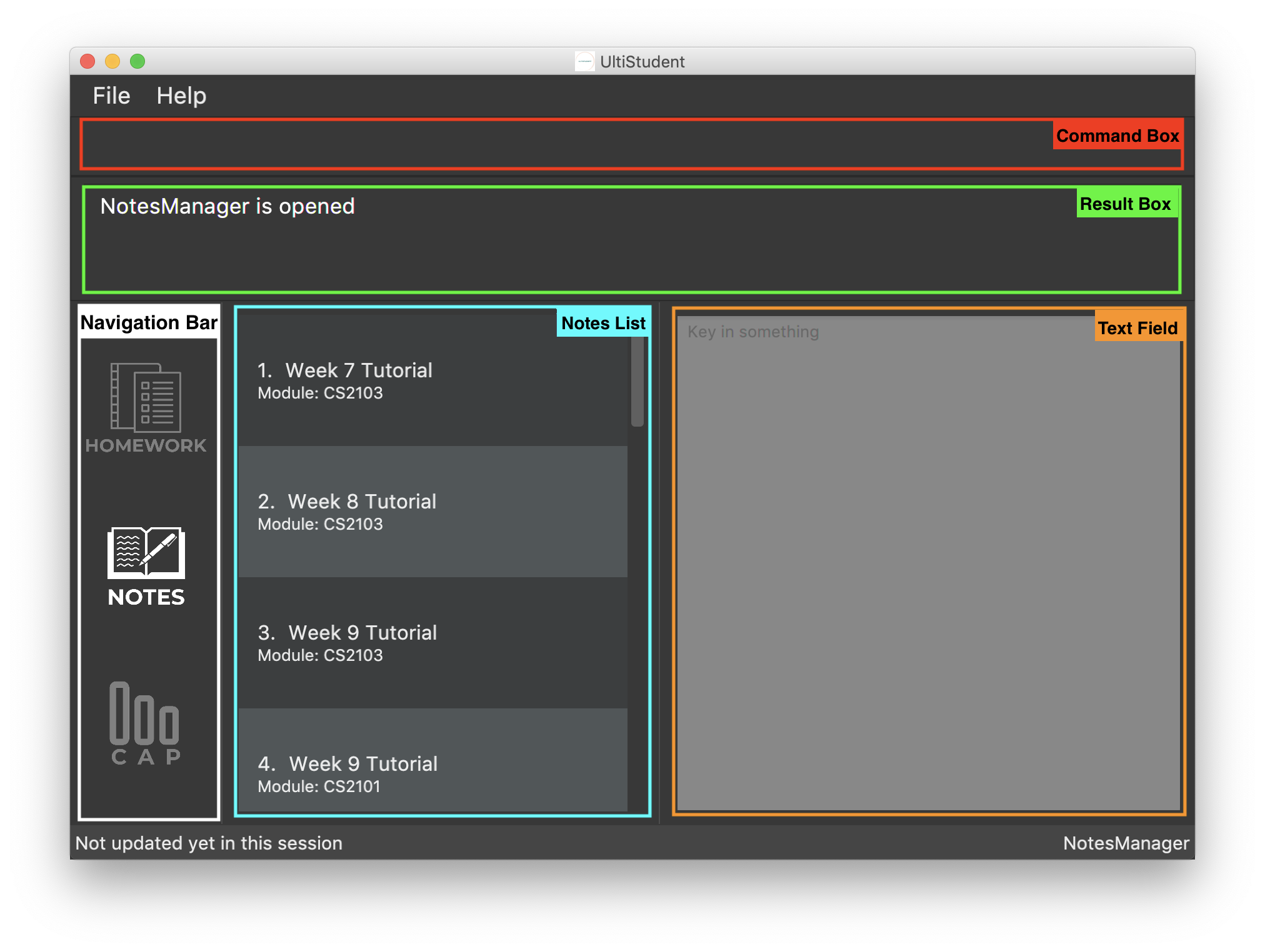
In this case, we need to add a new note. We will need to enter the following
command into the Command Box: add-note mc/CS2103T n/Week 7 Lecture
.
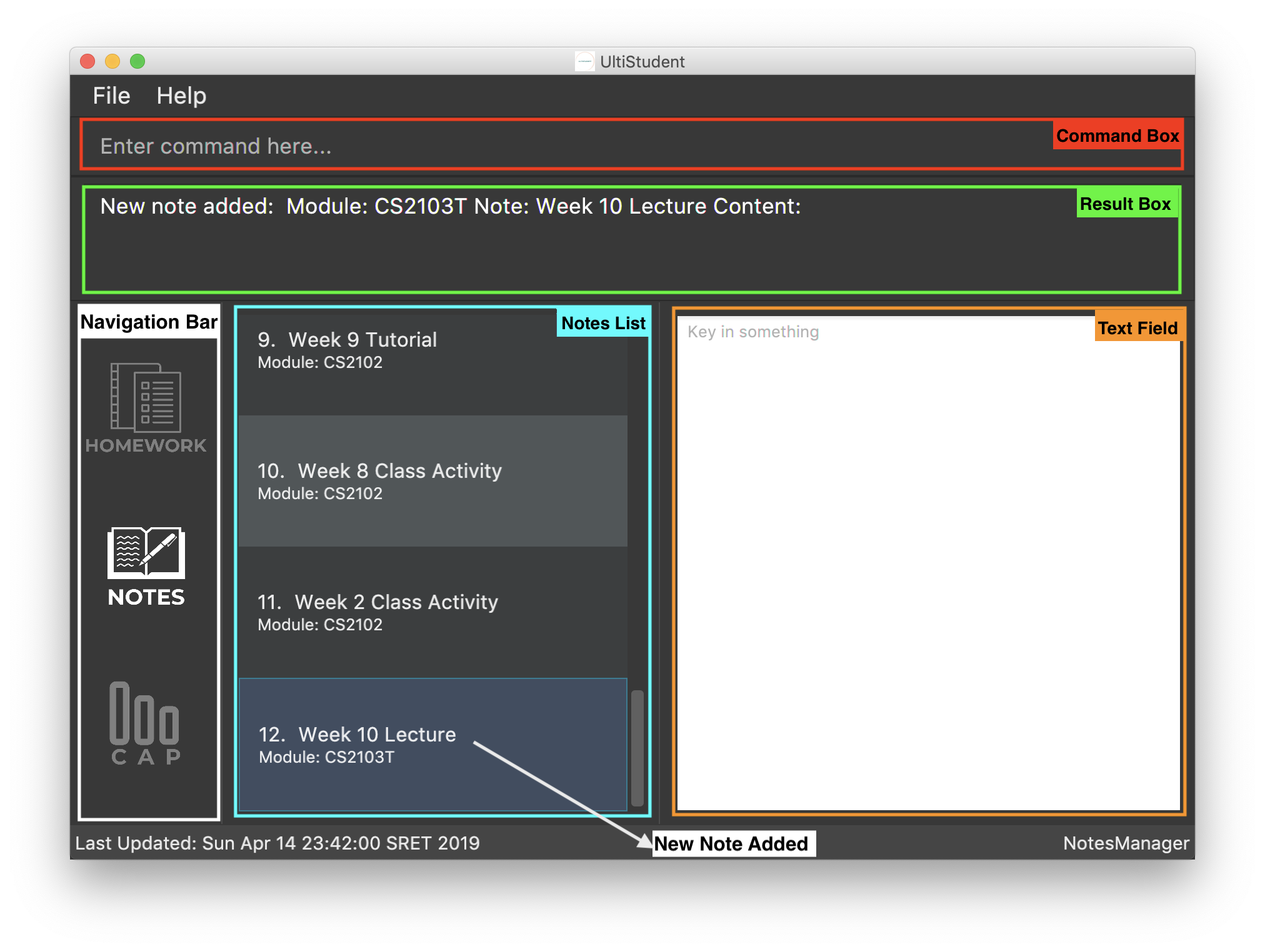
The new note will be added to the bottom of the list.
Notes Manager can only accept alphanumeric characters (A-Z, a-z, 0-9), and note names cannot begin with a whitespace. |
3.2. Deleting a Note: delete-note
Format: delete-note
As the semester comes to an end, we would like to clean up the notes by
removing some of the notes which we no longer need. Here’s where
delete-note
comes in handy!
To use this command we have to follow this format: delete-note INDEX
. This
will delete the note at the specified index. The index must be a positive
integer (1, 2, 3,…) and it must refer to an existing index number shown in
the displayed Notes List.
Say, we would like to delete the note we added from CS2103T Week 7 Lecture.
It has an index of 12
in the list.
As such, we will need to enter the following command: delete-note 12
.
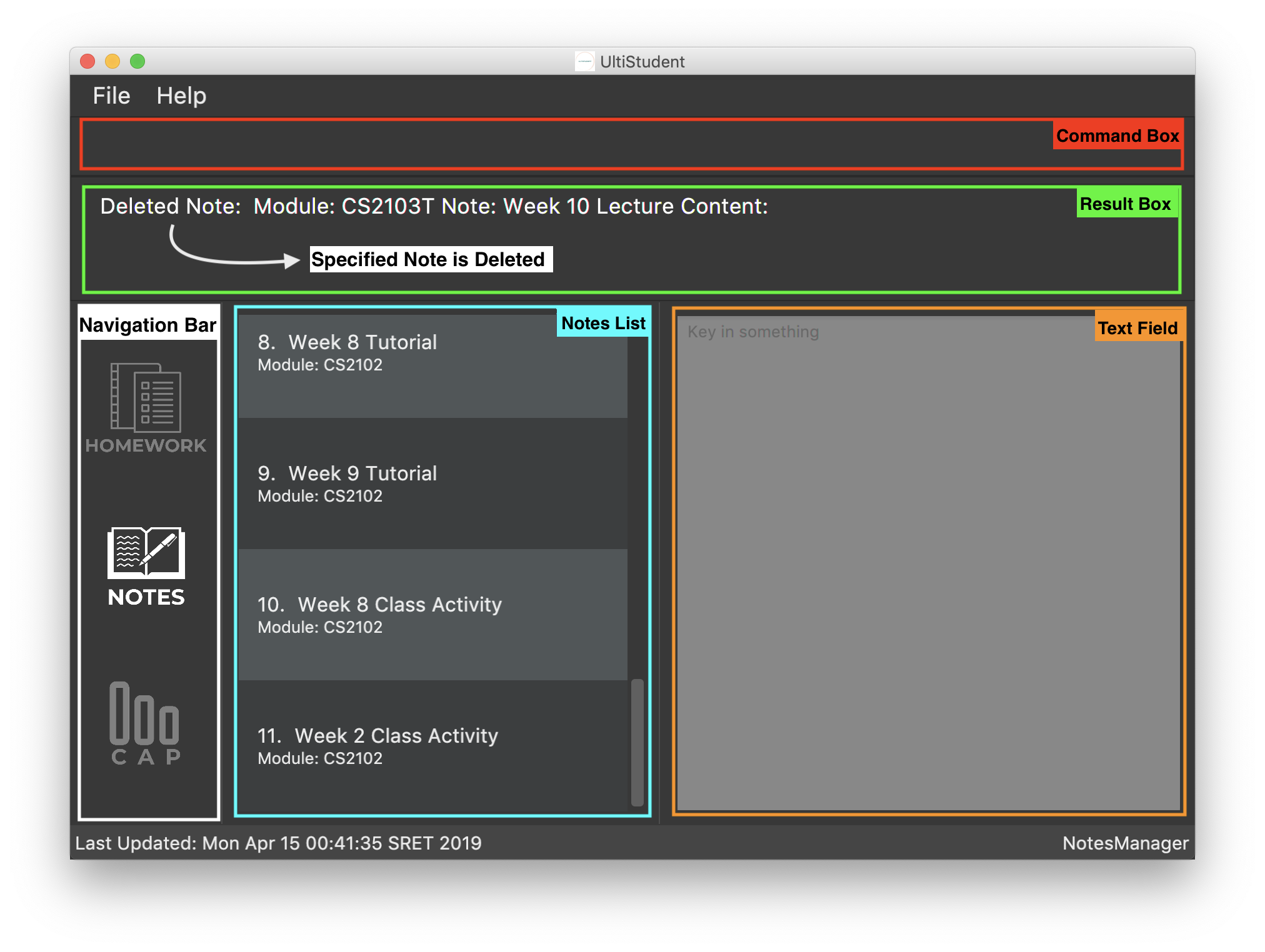
Viola! The note is deleted.
4. Contributions to the Developer Guide
This section is to showcase the technical depth of my contribution to UltiStudent, and highlight my ability to write technical documentation targeting developers.
4.1. Adding a note : add-note
The add homework feature is a core feature to the Homework Manager of UltiStudent. Which allows the users to create a homework object in UltiStudent. |
4.1.1. Overview
The add note add-note
mechanism is facilitated by AddNoteCommand
and
AddNoteCommandParser
.
It takes in the following input from the user: ModuleCode
and NoteName
which will construct individual objects that construct a Note
object.
The AddNoteCommandParser
implements Parser
with the following operation:
-
AddNoteCommandParser#parse()
- This operation will take in aString
input from the user that will create individual objects based on the prefixes 'mc/' and 'n/'. TheString
value after the individual prefixes will create the respective object: 'mc/' forModuleCode
and 'n/' forNoteName
. A validation check will be imposed upon the creation of each object. Any checks that fails the validation would prompt the user on the failed component.
For example:
-
ModuleCode
would useParserUtil#parseNoteModuleCode
to ensure that user has input 2 letters followed by 4 digits with an option to include a optional letter after the 4 digits. -
NoteName
would useParserUtil#parseNoteName
to ensure that note name is not a null.-
After validation checks are completed with no errors, a
Note
object is then constructed withModuleCode
andNoteName
. -
AddNoteCommandParser
would then return aAddNoteCommand
object with theNote
as the parameter
-
4.1.2. Example
Given below is an example usage scenario of how add-note
mechanism behaves at
each step.
Step 1: The user executes `add-note mc/CS2103T n/Week 1 Lecture to add a note for CS2103T Week 1 Lecture.
Step 2: LogicManager
would use UltiStudentParser#parse()
to parse the input from the user.
Step 3: UltiStudentParser
would determine which command is being used and creates the respective
parser. In this case, AddNoteCommandParser is being created and the user’s
input would be pass in as a parameter.
Step 4: AddNoteCommandParser
would do a validation check on the user’s input
before creating and returning a AddNoteCommand
object with Note
as the parameter.
Step 5: LogicManager
would use AddNoteCommand#execute()
to add the
ModuleCode
and Note
into the Model
which is handled by ModelManager
Step 6: AddNoteCommand
would return a CommandResult
to the LogicManager
which would then be return back to the user.
The image below illustrates the scenario of when the user executes add-hw mc/CS1101S hw/Tutorial 1
d/01/05/2019
:
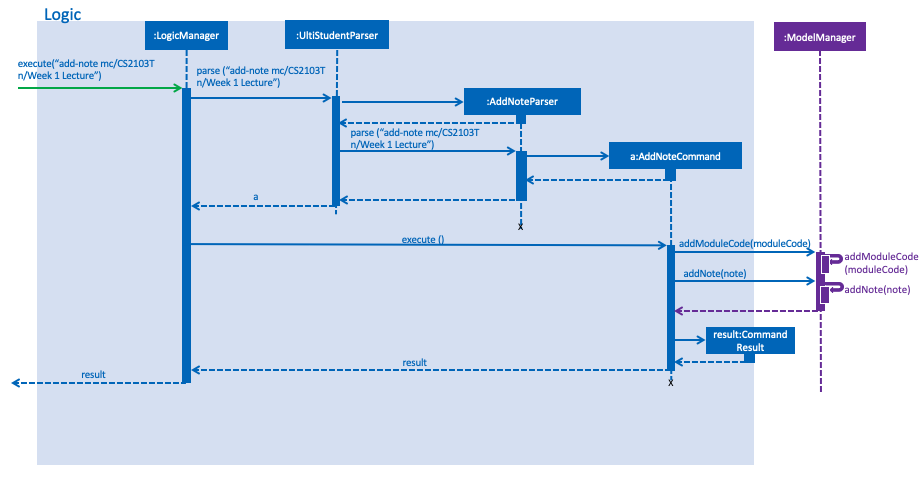
add-note
4.2. Deleting a note : delete-note
This feature allows the user to delete a note from the homework manager through its index.
The delete note feature is facilitated by the DeleteNoteCommandParser
and
the DeleteNoteCommand
.
The delete command is part of the logic component of our application. It interacts with the model and the storage components of our application.
4.2.1. Overview
The DeleteNoteCommandParser
implements Parser
with the following operation:
-
DeleteNoteCommandParser#parse()
- This operation will take in aint
input from the user which will delete the note entry at the index which has entered. Any invalid format of the command will be prompted by the command parser.
4.2.2. Current Implementation
The delete note feature is executed by the DeleteNoteCommand
. Currently,
the deletion of any note entry is done based on the INDEX
of the
note entry.
During the design of our delete function, we considered between two alternatives.
Description of Implementation | Pros and Cons |
---|---|
Deletion by Index (Current Choice) |
Pros: Since each note has a unique index within each list, deleting a note by its index is less prone to bugs and will be easier to implement. Cons: User will need to scroll for the entry and look for its index which can be inconvenient. |
Deletion by Homework Name |
Pros: It may be more intuitive for users to delete a note through its note name. Cons: Notes for different modules can have similar names. For example, two different note entries for two different modules can be called 'Week 7 Lecture'. This may cause it to be more error-prone. |
We have decided to opt for the first option primarily because it reduces the number of potential bugs and the complexities involved when taking into account the different cases upon using deletion by note name.